C# 8 中有几个比较好玩的新特性,比如下面的这两个:System.Index
和 System.Range
,分别对应着索引和切片操作,这篇文章将会讨论这两个类的使用。
System.Index 和 System.Range 结构体
可以用它们在运行时对集合进行 index
和 slice
,下面就是 System.Index
结构体的定义。.
namespace System
{
public readonly struct Index
{
public Index(int value, bool fromEnd);
}
}
然后就是 System.Range
结构体的定义。
namespace System
{
public readonly struct Range
{
public Range(System.Index start, System.Index end);
public static Range StartAt(System.Index start);
public static Range EndAt(System.Index end);
public static Range All { get; }
}
}
使用 System.Index 从尾部向前对集合进行索引
在 C# 8.0 之前没有任何方式可以从集合的尾部向前进行索引,现在你可以使用 ^
操作符实现对集合的从后往前索引,如下代码所示:
System.Index operator ^(int fromEnd);
接下来用一个例子来理解该操作符的使用,考虑下面的string数组。
string[] cities = { "Kolkata", "Hyderabad", "Bangalore", "London", "Moscow", "London", "New York" };
接下来的代码片段展示了如何使用 ^
运算符来获取 cities 集合的最后一个元素。
var city = cities[^1];
Console.WriteLine("The selected city is: " + city);
下面是完整的可供参考的代码:
public static void Main(string[] args)
{
string[] cities = { "Kolkata", "Hyderabad", "Bangalore", "London", "Moscow", "London", "New York" };
var city = cities[^1];
Console.WriteLine("The selected city is: " + city);
Console.ReadLine();
}
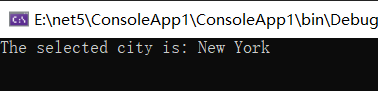
使用 System.Range 来提取子序列
你可以使用 System.Range
从 array 或者 span 类型上提取子集合,下面的代码展示了如何使用 range 和 index 来提取 string 的最后六个字符。
class Program
{
public static void Main(string[] args)
{
string str = "Hello World!";
Console.WriteLine(str[^6..]);
Console.ReadLine();
}
}
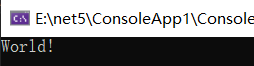
接下来是一个如何从 array 上提取子集合的例子。
public static void Main(string[] args)
{
int[] integers = { 0, 1, 2, 3, 4, 5, 6, 7, 8, 9 };
var slice = integers[1..5];
foreach (int i in slice)
{
Console.WriteLine(i);
}
Console.ReadLine();
}
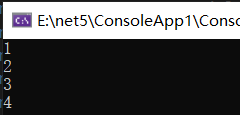
从图中可以看出,输出的数字为 1,2,3,4,即表示是一个 [)
的区间。
在 C#8 之前没有这样非常语义化的方式对集合进行 index 和 range,现在不一样了,你可以使用 ^
和 ..
这两个语法糖,让你的代码更加干净,可读,易维护。