你好,这里是 Dotnet 工具箱,定期分享 Dotnet 有趣,实用的工具或组件,希望对您有用!
简介
LiteDB 的灵感来自 MongoDB 数据库,所以它的 API 和 MongoDB 的 .NET API 非常相似。.
功能特性
• 无服务器 NoSQL 文档存储
• 类似于 MongoDB 的简洁 API
• 支持 .NET 4.5 / NETStandard 2.0
• 线程安全
• LINQ 查询的支持
• 具有完整事务支持的 ACID
• 单文件存储,类似于 SQLite
• 存储文件和流数据
• LiteDB Studio - 数据查询工具
• 开源免费
1. 在项目中使用 Nuget 安装 LiteDB。
2. 创建实体类
public class Customer
{
public int Id { get; set; }
public string Name { get; set; }
public string[] Phones { get; set; }
public bool IsActive { get; set; }
}
3. 打开数据库,如果不存在会自动创建。
using var db = new LiteDatabase(@"MyData.db");
4. 下面是一个增删改查的例子。
// 获取 Customers 集合
var col = db.GetCollection<Customer>("customers");
// 创建一个对象
var customer = new Customer
{
Name = "John Doe",
Phones = new string[] { "8000-0000", "9000-0000" },
Age = 39,
IsActive = true
};
// 在 Name 字段上创建唯一索引
col.EnsureIndex(x => x.Name, true);
// 数据插入
col.Insert(customer);
// 数据查询
List<Customer> list = col.Find(x => x.Age > 20).ToList();
Customer user = col.FindOne(x => x.Age > 20);
// 数据删除
col.Delete(user.Id);
另外LiteDB 还支持存储文件。
var storage = db.GetStorage<int>();
// 上传文件
storage.Upload(123, @"C:\Temp\picture-01.jpg");
// 下载文件
storage.Download(123, @"C:\Temp\copy-of-picture-01.jpg");
数据查询 - LiteDB.Studio
LiteDB.Studio 是一个用来查看和编辑 LiteDB 数据的 GUI 工具,并且支持 SQL 命令。
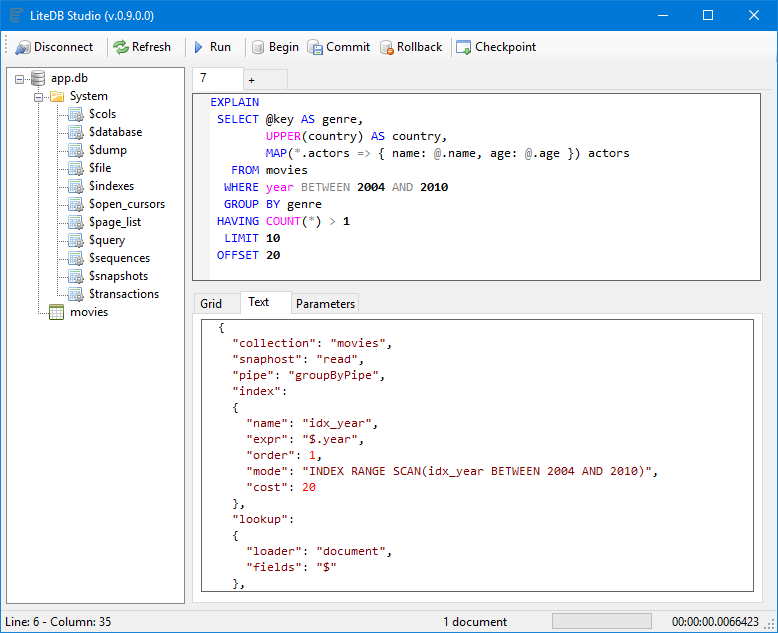