曾经也实现过.Net Framework 基于AppDomain 的 dll库热插拔,经历了版本的迭代,.Net Core 不支持 AppDomain,之前也搞过.Net Core 3.1 版本的,现在搞一下子.NET 6.0的。.
热插拔运用的场景
主要运用到宿主与插件这个场景或者动态任务的场景上(假设你现在业务服务已经运行,但是,需要新增加新的业务功能,就可以用这种方式)。
就像Office 或者 Visual Studio 一样,它们都是集插件架构之大成者。
逻辑实现
主要是根据 AssemblyLoadContext 这个系统提供的API来实现的,已经实现了对DLL程序集的加载和卸载。
之前AppDomain是通过程序域(隔离的环境)的概念进行隔离的,而 AssemblyLoadContext 的话,提供了程序集加载隔离,它允许在单个进程中加载同一程序集的多个版本。
它替换.NET Framework中多个AppDomain实例提供的隔离机制,其中AssemblyLoadContext.Default 表示运行时的默认上下文,该上下文用于应用程序主程序集及其静态依赖项,那么,其他的上下文,就是插件DLL的上下文了。
从概念上讲,加载上下文会创建一个用于加载、解析和可能卸载一组程序集的范围。
这里就根据 AssemblyLoadContext 加载,卸载,来实现热插播逻辑的实现.
实现逻辑
主要的逻辑是这个逻辑
/// <summary>
/// dll文件的加载
/// </summary>
public class LoadDll
{
/// <summary>
/// 任务实体
/// </summary>
public ITask _task;
public Thread _thread;
/// <summary>
/// 核心程序集加载
/// </summary>
public AssemblyLoadContext _AssemblyLoadContext { get; set; }
/// <summary>
/// 获取程序集
/// </summary>
public Assembly _Assembly { get; set; }
/// <summary>
/// 文件地址
/// </summary>
public string filepath = string.Empty;
/// <summary>
/// 指定位置的插件库集合
/// </summary>
AssemblyDependencyResolver resolver { get; set; }
public bool LoadFile(string filepath)
{
this.filepath = filepath;
try
{
resolver = new AssemblyDependencyResolver(filepath);
_AssemblyLoadContext = new AssemblyLoadContext(Guid.NewGuid().ToString("N"), true);
_AssemblyLoadContext.Resolving += _AssemblyLoadContext_Resolving;
using (var fs = new FileStream(filepath, FileMode.Open, FileAccess.Read))
{
var _Assembly = _AssemblyLoadContext.LoadFromStream(fs);
var Modules = _Assembly.Modules;
foreach (var item in _Assembly.GetTypes())
{
if (item.GetInterface("ITask") != null)
{
_task = (ITask)Activator.CreateInstance(item);
break;
}
}
return true;
}
}
catch (Exception ex) { Console.WriteLine($"LoadFile:{ex.Message}"); };
return false;
}
private Assembly _AssemblyLoadContext_Resolving(AssemblyLoadContext arg1, AssemblyName arg2)
{
Console.WriteLine($"加载{arg2.Name}");
var path = resolver.ResolveAssemblyToPath(arg2);
if (!string.IsNullOrEmpty(path))
{
using (var fs = new FileStream(path, FileMode.Open, FileAccess.Read))
{
return _AssemblyLoadContext.LoadFromStream(fs);
}
}
return null;
}
public bool StartTask()
{
bool RunState = false;
try
{
if (_task != null)
{
_thread = new Thread(new ThreadStart(_Run));
_thread.IsBackground = true;
_thread.Start();
RunState = true;
}
}
catch (Exception ex) { Console.WriteLine($"StartTask:{ex.Message}"); };
return RunState;
}
private void _Run()
{
try
{
_task.Run();
}
catch (Exception ex) { Console.WriteLine($"_Run 任务中断执行:{ex.Message}"); };
}
public bool UnLoad()
{
try
{
_thread?.Interrupt();
}
catch (Exception ex)
{
Console.WriteLine($"UnLoad:{ex.Message}");
}
finally
{
_thread = null;
}
_task = null;
try
{
_AssemblyLoadContext?.Unload();
}
catch (Exception)
{ }
finally
{
_AssemblyLoadContext = null;
GC.Collect();
GC.WaitForPendingFinalizers();
}
return true;
}
}
以上就是这个热插拔的核心逻辑了。
ITask.cs
这个接口实现简单,只有一个方法,当然,如果有需要,可以扩展一下。
/// <summary>
/// 任务接口
/// </summary>
public interface ITask
{
/// <summary>
/// 任务的运行方法
/// </summary>
/// <returns></returns>
void Run();
}
插件库1 PrintStrLib
插件的代码就很简单了
public class PrintStr : ITask
{
public void Run()
{
int a = 0;
while (true)
{
Console.WriteLine($"PrintStr:{a}");
a++;
Thread.Sleep(1 * 1000);
}
}
}
插件库2 PrintDateLib
插件的代码就很简单了
public class PrintDate : ITask
{
public void Run()
{
while (true)
{
Console.WriteLine($"PrintDate:{DateTime.Now}");
Thread.Sleep(1 * 1000);
}
}
}
测试运行
使用也很简单,加载程序集,然后,执行,间隔指定时间后,顺序卸载。
static void Main(string[] args)
{
Console.Title = "AssemblyLoadContext Dll热插拔 测试 by 蓝总创精英团队";
var list = new List<LoadDll>();
Console.WriteLine("开始加载DLL");
list.Add(Load(Path.Combine(AppDomain.CurrentDomain.BaseDirectory,"DLL", "PrintDateLib.dll")));
list.Add(Load(Path.Combine(AppDomain.CurrentDomain.BaseDirectory, "DLL", "PrintStrLib.dll")));
foreach (var item in list)
{
item.StartTask();
}
Console.WriteLine("开启了任务!");
SpinWait.SpinUntil(() => false, 5 * 1000);
foreach (var item in list)
{
var s = item.UnLoad();
SpinWait.SpinUntil(() => false, 2 * 1000);
Console.WriteLine($"任务卸载:{s}");
}
Console.WriteLine("热插拔插件任务 测试完毕");
Console.ReadLine();
}
public static LoadDll Load(string filePath)
{
var load = new LoadDll();
load.LoadFile(filePath);
return load;
}
效果查看
从下图来看,我们想要的结果都有了,加载两个插件,插件执行自己的业务,然后,顺序一个一个的卸载掉,确实已经不在执行它自己的业务了。
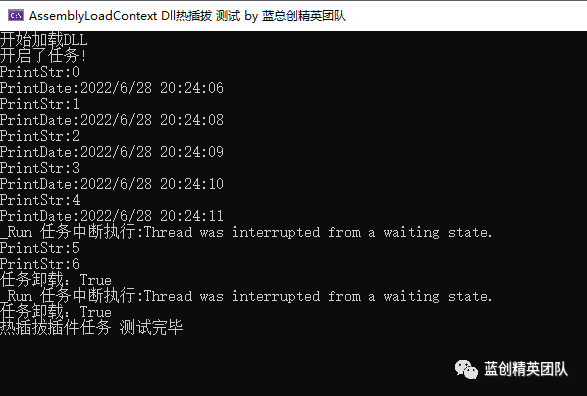
总结
实际上.Net的程序集的隔离问题很多,这种隔离方式实际用的过程中,如果程序集简单还好,复杂的话,可能会有别的问题。
我非常喜欢的隔离方式就像谷歌游览器那样的插件方式或者IIS那样的容器级隔离,不过,这种实际上我分析是进程级隔离方案,现在也流行docker,系统级隔离。
只能说存在即合理吧,有它存在的价值。
代码地址
https://github.com/kesshei/AssemblyLoadContextDemo.git
https://gitee.com/kesshei/AssemblyLoadContextDemo.git