前言
在上一篇文档中说到使用 IHostedService 接口实现定时任务传送门:https://www.cnblogs.com/ysmc/p/16456787.html,其中,有小伙伴就问到,为什么不使用 BackgroundService,我个人觉得使用什么技术,应该取决于需求,代码只是一种工具,用得顺手对于编码人员来说,我个人感觉还是非常重要的;正好也说到了 BackgroundService,那这一篇文档就简单说一下它吧。.
正文
首先我们看一下官方的说明,学习代码一定要看官方的文档,尽管有时候会有点晦涩难懂,但肯定是最正确的:
BackgroundService 基类
BackgroundService 是用于实现长时间运行的 IHostedService 的基类。
调用 ExecuteAsync(CancellationToken) 来运行后台服务。实现返回一个 Task,其表示后台服务的整个生存期。
在 ExecuteAsync 变为异步(例如通过调用 await
)之前,不会启动任何其他服务。避免在 ExecuteAsync
中执行长时间的阻塞初始化工作。
StopAsync(CancellationToken) 中的主机块等待完成 ExecuteAsync
。
调用 IHostedService.StopAsync 时,将触发取消令牌。当激发取消令牌以便正常关闭服务时,ExecuteAsync
的实现应立即完成。否则,服务将在关闭超时后不正常关闭。
StartAsync
应仅限于短期任务,因为托管服务是按顺序运行的,在 StartAsync
运行完成之前不会启动其他服务。长期任务应放置在 ExecuteAsync
中。
针对第一点“BackgroundService 是用于实现长时间运行的 IHostedService 的基类”,我们先看看 BackgroundService 的源码:
public abstract class BackgroundService : IHostedService, IDisposable
{
private Task _executingTask;
private readonly CancellationTokenSource _stoppingCts = new CancellationTokenSource();
/// <summary>
/// This method is called when the <see cref="IHostedService"/> starts. The implementation should return a task that represents
/// the lifetime of the long running operation(s) being performed.
/// /// </summary>
/// <param name="stoppingToken">Triggered when <see cref="IHostedService.StopAsync(CancellationToken)"/> is called.</param>
/// <returns>A <see cref="Task"/> that represents the long running operations.</returns>
protected abstract Task ExecuteAsync(CancellationToken stoppingToken);
/// <summary>
/// Triggered when the application host is ready to start the service.
/// </summary>
/// <param name="cancellationToken">Indicates that the start process has been aborted.</param>
public virtual Task StartAsync(CancellationToken cancellationToken)
{
// Store the task we're executing
_executingTask = ExecuteAsync(_stoppingCts.Token);
// If the task is completed then return it, this will bubble cancellation and failure to the caller
if (_executingTask.IsCompleted)
{
return _executingTask;
}
// Otherwise it's running
return Task.CompletedTask;
}
/// <summary>
/// Triggered when the application host is performing a graceful shutdown.
/// </summary>
/// <param name="cancellationToken">Indicates that the shutdown process should no longer be graceful.</param>
public virtual async Task StopAsync(CancellationToken cancellationToken)
{
// Stop called without start
if (_executingTask == null)
{
return;
}
try
{
// Signal cancellation to the executing method
_stoppingCts.Cancel();
}
finally
{
// Wait until the task completes or the stop token triggers
await Task.WhenAny(_executingTask, Task.Delay(Timeout.Infinite, cancellationToken));
}
}
public virtual void Dispose()
{
_stoppingCts.Cancel();
}
}
以上代码很好的解答了小伙伴提出“为什么不使用 BackgroundService”的问题,在上一篇文章中,评论区的一位大佬也很好的回答了这位小伙伴的问题,我这里引用下这位大佬的原话:“BackgroundService 是 IHostedService的一个简单实现,内部IHostedService 的StartAsync调用了ExecuteAsync”,本质上就是使用了 IHostedService;
让我们回到正题,怎么用 BackgroundService 实现定时任务呢,老规矩,上代码:
首先,创建一个服务接口,定义需要实现的任务,以及对应的实现,如果需要执行异步方法,记得加上 await,不然任务将不会等待执行结果,直接进行下一个任务。
public class TaskWorkService : ITaskWorkService
{
public async Task TaskWorkAsync(CancellationToken stoppingToken)
{
while (!stoppingToken.IsCancellationRequested)
{
//执行任务
Console.WriteLine($"{DateTime.Now}");
//周期性任务,于上次任务执行完成后,等待5秒,执行下一次任务
await Task.Delay(500);
}
}
}
注册服务
builder.Services.AddScoped<ITaskWorkService, TaskWorkService>();
创建后台服务类,继承基类 BackgroundService,这里需要注意的是,要在 BackgroundService 中使用有作用域的服务,请创建作用域, 默认情况下,不会为托管服务创建作用域,得自己管理服务的生命周期,切记!于构造函数中注入 IServiceProvider即可。
public class BackgroundServiceDemo : BackgroundService
{
private readonly IServiceProvider _services;
public BackgroundServiceDemo(IServiceProvider services)
{
_services = services;
}
protected override async Task ExecuteAsync(CancellationToken stoppingToken)
{
using var scope = _services.CreateScope();
var taskWorkService = scope.ServiceProvider.GetRequiredService<ITaskWorkService>();
await taskWorkService.TaskWorkAsync(stoppingToken);
}
}
最后别忘了这个类也是需要注册的,注册方式与 IHostedService 接口的方式一样
builder.Services.AddHostedService<BackgroundServiceDemo>();
大功告成,F5看看效果吧
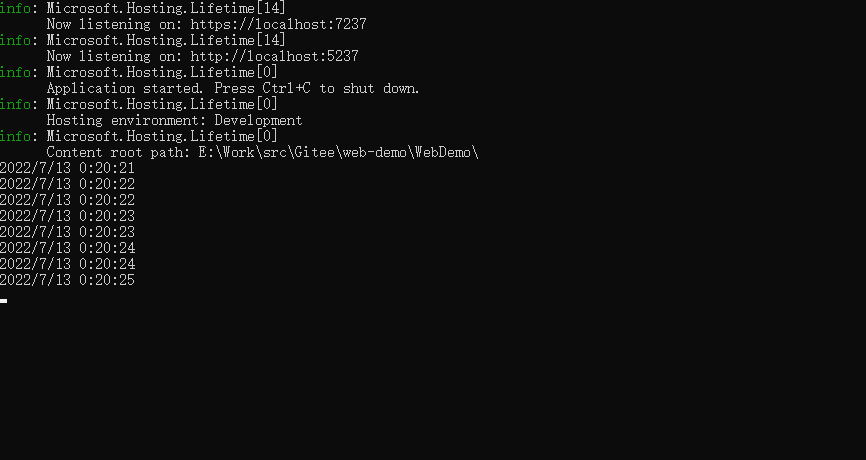
最后
Bootstrap Blazor官网地址:https://www.blazor.zone,希望大佬们看到这篇文章,能给项目点个star支持下,感谢各位!