-
框架使用 .NET40
; -
Visual Studio 2019
; -
以下几种方案可以播放 rtsp
:-
Vlc -
Emgu -
WPFMediaKit
-
-
此篇是 WPFMediaKit
播放rtsp
视频流,再使用控件MediaUriElement
进行播放rtsp
时通常不会成功(可能会出现报错或者直接不显示画面),那是因为本地没有按照解码器导致。 -
WPF-MediaKit
要求在计算机上安装DirectShow
编解码器。 -
可以安装以下解码器解决无法播放 rtsp
视频流问题.-
K-Lite下载完成运行 -
LAVFilters -
ffdshow
-

-
安装完成后就可以正常预览 rtsp
视频流. -
创建项目后引入 Nuget
包。
1)新建 MainWindow.xaml
代码如下:
<wpfdev:Window x:Class="WPFPlayRTSPExample.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:wpfdev="https://github.com/WPFDevelopersOrg/WPFDevelopers"
xmlns:controls="clr-namespace:WPFMediaKit.DirectShow.Controls;assembly=WPFMediaKit"
xmlns:local="clr-namespace:WPFPlayRTSPExample"
mc:Ignorable="d" WindowStartupLocation="CenterScreen"
Title="WPFPlayRTSPExample" Height="450" Width="800">
<wpfdev:SixGirdView BorderThickness="1" SelectBrush="Red">
<wpfdev:SixGirdView.Resources>
<Style TargetType="TextBlock">
<Setter Property="Foreground" Value="White"/>
<Setter Property="VerticalAlignment" Value="Center"/>
<Setter Property="HorizontalAlignment" Value="Center"/>
</Style>
<Style TargetType="Border">
<Setter Property="Margin" Value="1"/>
</Style>
<Style TargetType="RadioButton">
<Setter Property="Margin" Value="1"/>
<Setter Property="Template">
<Setter.Value>
<ControlTemplate TargetType="RadioButton">
<wpfdev:SmallPanel>
<ContentPresenter HorizontalAlignment="{TemplateBinding HorizontalContentAlignment}"
Margin="{TemplateBinding Padding}"
VerticalAlignment="{TemplateBinding VerticalContentAlignment}"/>
<Border Background="{DynamicResource PrimaryNormalSolidColorBrush}"
VerticalAlignment="Top"
HorizontalAlignment="Left"
Padding="10,4"
CornerRadius="3"
Name="PART_Border"
Visibility="Collapsed">
<TextBlock Text="正在播放" Foreground="White"/>
</Border>
</wpfdev:SmallPanel>
<ControlTemplate.Triggers>
<Trigger Property="IsChecked" Value="True">
<Setter Property="Visibility" TargetName="PART_Border" Value="Visible"/>
</Trigger>
</ControlTemplate.Triggers>
</ControlTemplate>
</Setter.Value>
</Setter>
</Style>
</wpfdev:SixGirdView.Resources>
<controls:MediaUriElement x:Name="mediaUriElement" Margin="1"/>
<RadioButton DataContext="{Binding RtspModelCollection[0],RelativeSource={RelativeSource AncestorType=local:MainWindow}}"
Checked="RadioButton_Checked" x:Name="rBtn1">
<Image Source="/Images/grid1.png"/>
</RadioButton>
<RadioButton DataContext="{Binding RtspModelCollection[1],RelativeSource={RelativeSource AncestorType=local:MainWindow}}"
Checked="RadioButton_Checked">
<Image Source="/Images/grid2.png"/>
</RadioButton>
<RadioButton DataContext="{Binding RtspModelCollection[2],RelativeSource={RelativeSource AncestorType=local:MainWindow}}"
Checked="RadioButton_Checked">
<Image Source="/Images/grid3.png"/>
</RadioButton>
<RadioButton DataContext="{Binding RtspModelCollection[3],RelativeSource={RelativeSource AncestorType=local:MainWindow}}"
Checked="RadioButton_Checked">
<Image Source="/Images/grid4.png"/>
</RadioButton>
<Border Background="#282C34">
<TextBlock Text="无信号"/>
</Border>
</wpfdev:SixGirdView>
</wpfdev:Window>
2) MainWindow.xaml.cs
代码如下:
using System;
using System.Collections.ObjectModel;
using System.Windows;
using System.Windows.Controls;
namespace WPFPlayRTSPExample
{
/// <summary>
/// Interaction logic for MainWindow.xaml
/// </summary>
public partial class MainWindow
{
public ObservableCollection<RtspModel> RtspModelCollection
{
get { return (ObservableCollection<RtspModel>)GetValue(RtspModelCollectionProperty); }
set { SetValue(RtspModelCollectionProperty, value); }
}
public static readonly DependencyProperty RtspModelCollectionProperty =
DependencyProperty.Register("RtspModelCollection", typeof(ObservableCollection<RtspModel>), typeof(MainWindow), new PropertyMetadata(null));
public MainWindow()
{
InitializeComponent();
Loaded += delegate
{
RtspModelCollection = new ObservableCollection<RtspModel>();
RtspModelCollection.Add(new RtspModel { RTSPUri = "rtsp://wowzaec2demo.streamlock.net/vod/mp4:BigBuckBunny_115k.mp4" });
RtspModelCollection.Add(new RtspModel { RTSPUri = "http://devimages.apple.com/iphone/samples/bipbop/gear1/prog_index.m3u8" });
RtspModelCollection.Add(new RtspModel { RTSPUri = "http://ws.flv.huya.com/src/1394575534-1394575534-5989656310331736064-2789274524-10057-A-0-1.flv?wsSecret=cf05e7dbacda9cf2ec112517a276458c&wsTime=639bf822&fm=RFdxOEJjSjNoNkRKdDZUWV8kMF8kMV8kMl8kMw%3D%3D&ctype=huya_live&txyp=o%3An6%3B&fs=bgct&sphdcdn=al_7-tx_3-js_3-ws_7-bd_2-hw_2&sphdDC=huya&sphd=264_*-265_*&exsphd=264_500,264_2000,&ratio=500" });
RtspModelCollection.Add(new RtspModel { RTSPUri = "rtsp://admin:Ancn1111@192.168.21.128:554/h264/ch1/main/av_stream" });
rBtn1.IsChecked = true;
};
}
private void RadioButton_Checked(object sender, RoutedEventArgs e)
{
if(sender is RadioButton radio)
{
var model = radio.DataContext as RtspModel;
if (model == null) return;
mediaUriElement.Source = new Uri(model.RTSPUri);
}
}
}
public class RtspModel
{
public string RTSPUri { get; set; }
}
}
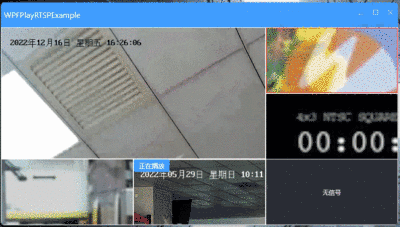