一、什么是MQTT?
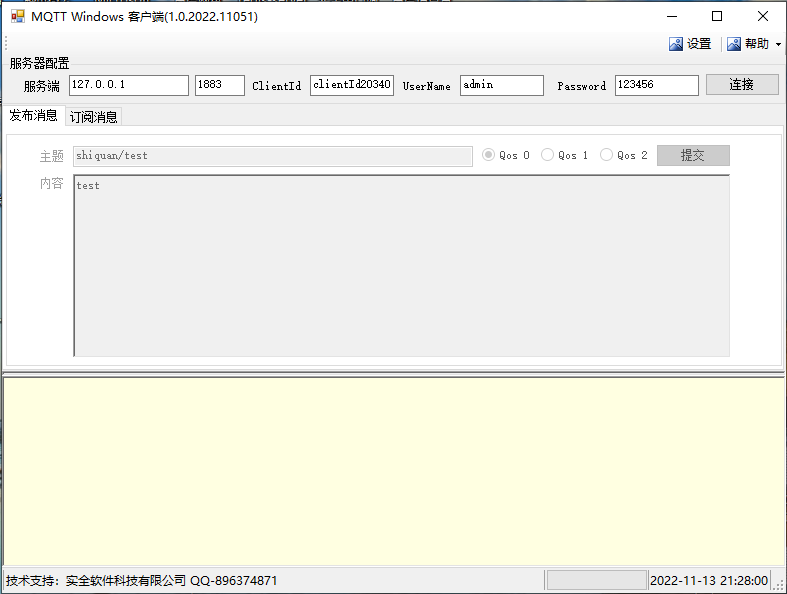
二、连接服务端
var option = new MQTT.MQTTClientOption()
{
ClientId = this.txtClientId.Text,
IpString = this.txtServer.Text,
Port = Convert.ToInt32(this.txtPort.Text),
UserName = this.txtUserName.Text,
Password = this.txtPassword.Text
};
//实例客户端
this.mqttClient = new MQTT.MQTTClient();
this.mqttClient.ClientReceived += MqttClient_ClientReceived;
var result = await this.mqttClient.ConnectAsync(option);
if(result.State == false)
{
MessageBox.Show(result.Fail, "提示", MessageBoxButtons.OK, MessageBoxIcon.Warning);
return;
}
//开始接收
this.mqttClient.BeginReceive();
三、发布消息
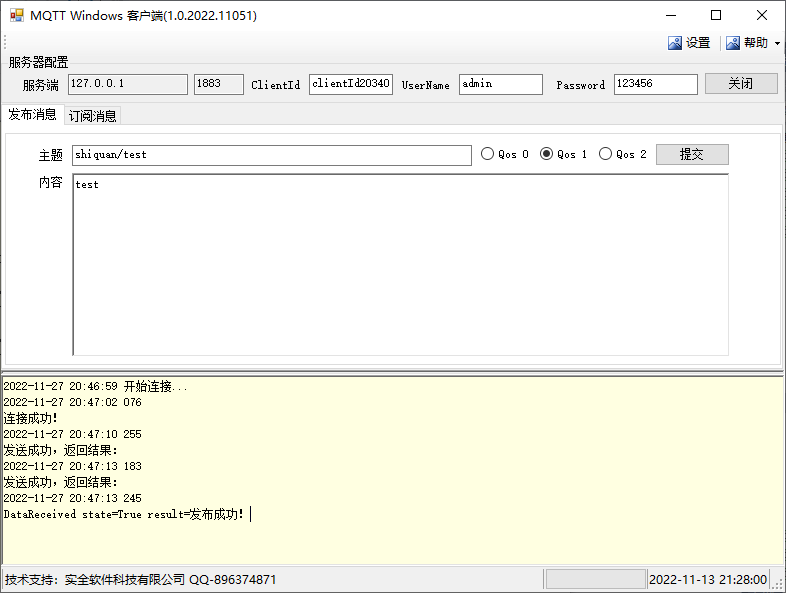
private async void BtnPublish_Click(object sender, EventArgs e)
{
this.btnPublish.Enabled = false;
this.Cursor = Cursors.WaitCursor;
try
{
short qos = 0;
if (rdbLevel1.Checked)
qos = 1;
if (rdbLevel2.Checked)
qos = 2;
var data = new MQTT.PublishEntity()
{
Topic = this.txtTopic.Text,
Reload = System.Text.Encoding.UTF8.GetBytes(this.txtContent.Text),
Qos = qos,
PacketId=_PacketId
};
var result = await this.mqttClient.PublishAsync(data,false);
if(result.State == false)
{
this.AppendText("发送失败:" + result.Fail);
return;
}
this._PacketId++;
this.AppendText("发送成功,返回结果:" + result.Result);
}
finally
{
this.btnPublish.Enabled = true;
this.Cursor = Cursors.Default;
}
}
四、订阅消息
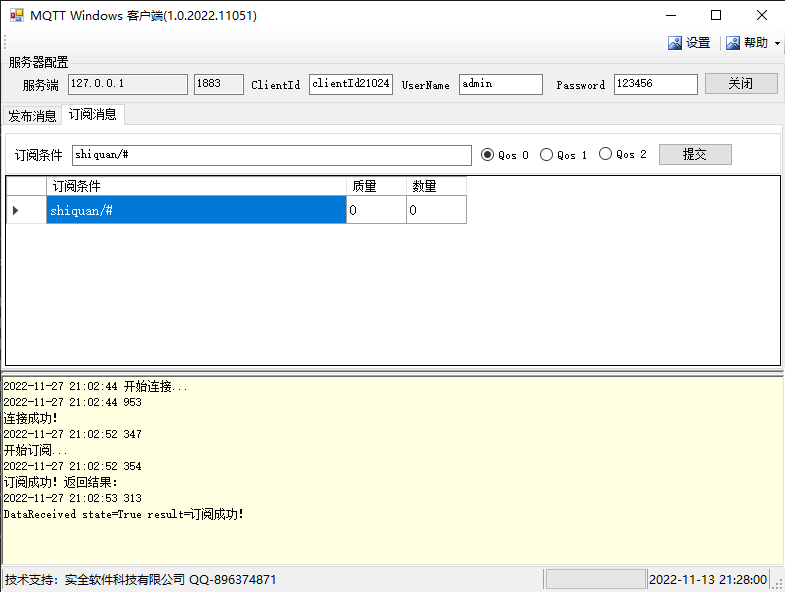
{
this.btnSubscribe.Enabled = false;
this.Cursor = Cursors.WaitCursor;
try
{
string fail = string.Empty;
short qos = 0;
if (rdbQos1.Checked)
qos = 1;
if (rdbQos2.Checked)
qos = 2;
MQTT.SubscribeEntity entity = new MQTT.SubscribeEntity();
entity.Filter = this.txtFilter.Text;
entity.Qos = qos;
this.AppendText("开始订阅...");
//if (mqttClient.Subscribe(this.txtFilter.Text, qos, out result, out fail) == false)
//{
// this.AppendText(fail);
// return;
//}
var result = await mqttClient.SubscribeAsync(entity.Filter,entity.Qos);
if (result.State == false)
{
this.AppendText(result.Fail);
return;
}
this.AppendText("订阅成功!返回结果:" + result.Result);
MQTT.SubscribeHelper.Save(entity);
DataRow drData = this.dtSubscribes.NewRow();
drData["Id"] = entity.Id;
drData["Filter"] = entity.Filter;
drData["Qos"] = entity.Qos;
drData["Count"] = 0;
dtSubscribes.Rows.Add(drData);
}
finally
{
this.btnSubscribe.Enabled = true;
this.Cursor = Cursors.Default;
}
}
五、取消订阅
客户端取消某一订阅消息。双击订阅消息行,取消订阅消息。
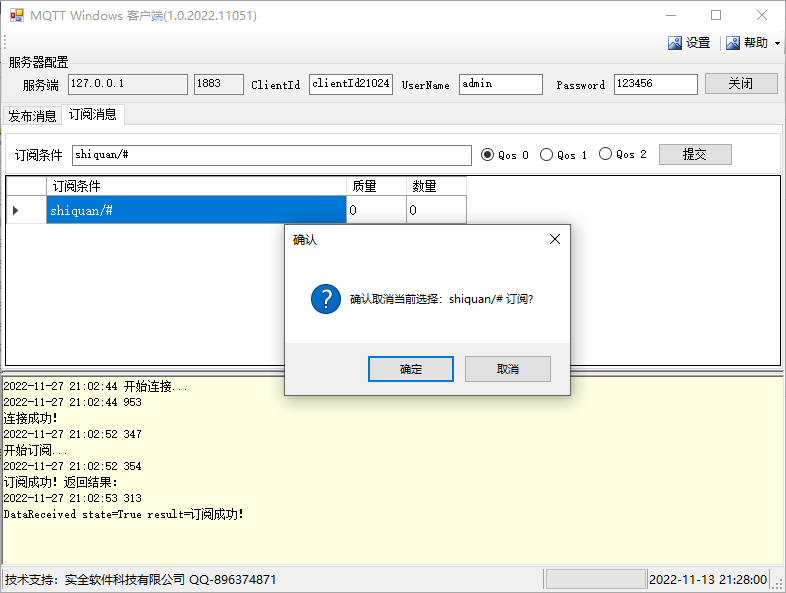
{
if (this.dataGridView1.CurrentCell == null)
return;
DataRowView drv = this.dataGridView1.CurrentRow.DataBoundItem as DataRowView;
if (drv == null)
return;
if (MessageBox.Show("确认取消当前选择:" + drv["Filter"].ToString() + " 订阅?", "确认", MessageBoxButtons.OKCancel, MessageBoxIcon.Question) != DialogResult.OK)
return;
try
{
this.AppendText("开始取消订阅...");
var result = await mqttClient.UnSubscribeAsync(drv["Filter"].ToString());
if (result.State == false)
{
this.AppendText(result.Fail);
return;
}
this.AppendText("取消订阅成功!返回结果:" + result.Result);
MQTT.SubscribeHelper.Delete(drv["Id"].ToString());
this.dataGridView1.Rows.RemoveAt(this.dataGridView1.CurrentCell.RowIndex);
}
catch (Exception ex)
{
this.AppendText("取消订阅异常:" + ex.ToString());
}
}
六、接收服务端消息
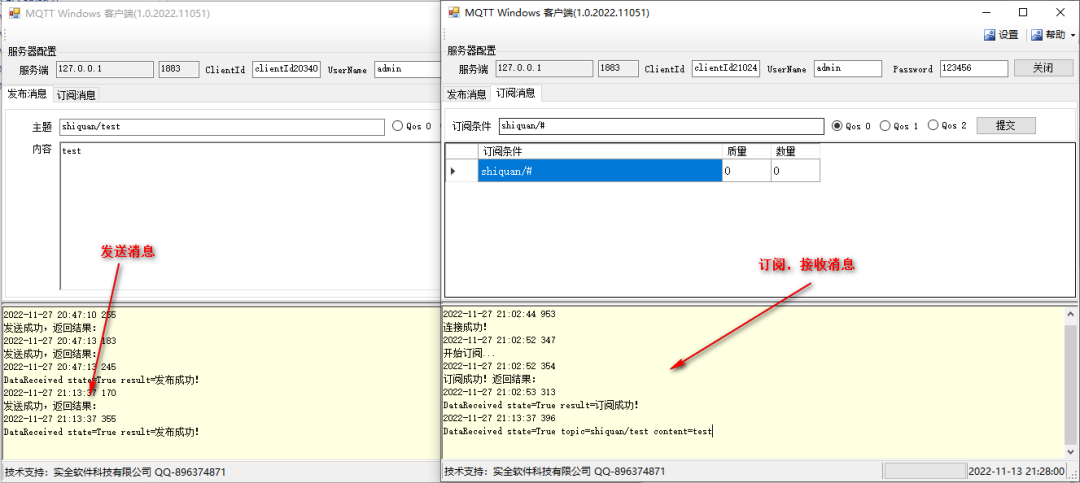
{
if(receive.Data != null)
this.AppendText("DataReceived state="+receive.State+" topic="+ receive.Data.Topic + " content=" + System.Text.Encoding.UTF8.GetString(receive.Data.Reload));
else if(receive.State)
this.AppendText("DataReceived state=" + receive.State + " result=" + receive.Result);
else
this.AppendText("DataReceived state=" + receive.State + " fail=" + receive.Fail);
}