概述
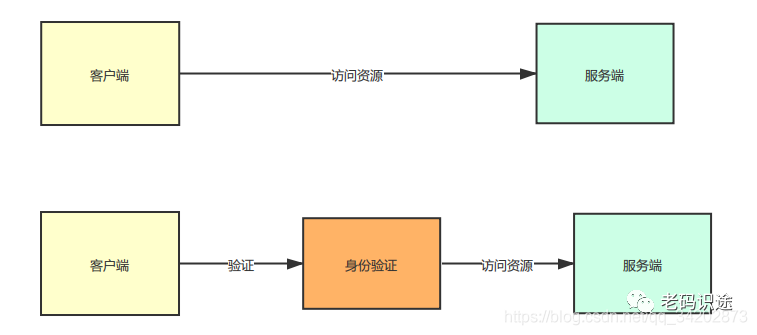
什么是鉴权,授权?
-
对用户进行身份验证。
-
在未经身份验证的用户试图访问受限资源时作出响应。
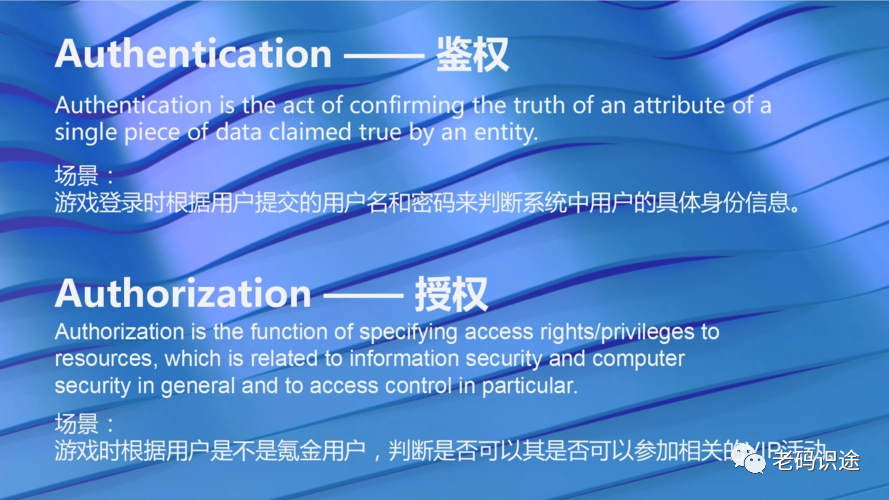
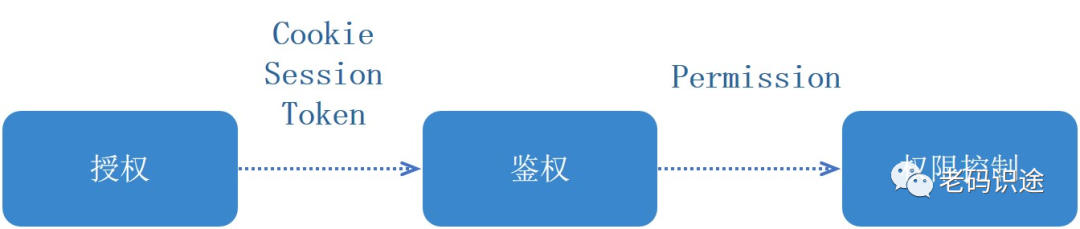
鉴权授权基本步骤
//添加鉴权服务
builder.Services.AddAuthentication(options =>
{
options.DefaultScheme = CookieAuthenticationDefaults.AuthenticationScheme;
}).AddCookie(CookieAuthenticationDefaults.AuthenticationScheme, options =>
{
options.LoginPath = "/Login/Index";
options.LogoutPath = "/Login/Logout";
});
//使用鉴权
app.UseAuthentication();
//使用授权
app.UseAuthorization();
namespace DemoCoreMVC.Controllers
{
[Authorize]
public class HomeController : Controller
{
private readonly ILogger<HomeController> _logger;
public HomeController(ILogger<HomeController> logger)
{
_logger = logger;
}
public IActionResult Index()
{
var username = HttpContext.Session.GetString("username");
ViewBag.Username = username;
return View();
}
public IActionResult Privacy()
{
return View();
}
}
}
namespace DemoCoreMVC.Controllers
{
public class LoginController : Controller
{
public IActionResult Index()
{
return View();
}
public async Task<IActionResult> Login()
{
var username = Request.Form["username"];
var password = Request.Form["password"];
if(username=="admin" && password == "abc123")
{
HttpContext.Session.SetString("username", username);
}
var claimsIdentity = new ClaimsIdentity(CookieAuthenticationDefaults.AuthenticationScheme);
claimsIdentity.AddClaim(new Claim(ClaimTypes.Name,username));
claimsIdentity.AddClaim(new Claim(ClaimTypes.Role,"Admin"));
var claimPrincipal = new ClaimsPrincipal(claimsIdentity);
await HttpContext.SignInAsync(claimPrincipal);
return Redirect("/Home");
}
public async Task<IActionResult> Logout()
{
await HttpContext.SignOutAsync();
return Redirect("/Login");
}
}
}
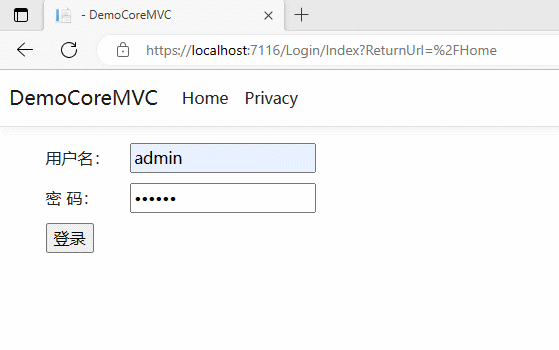
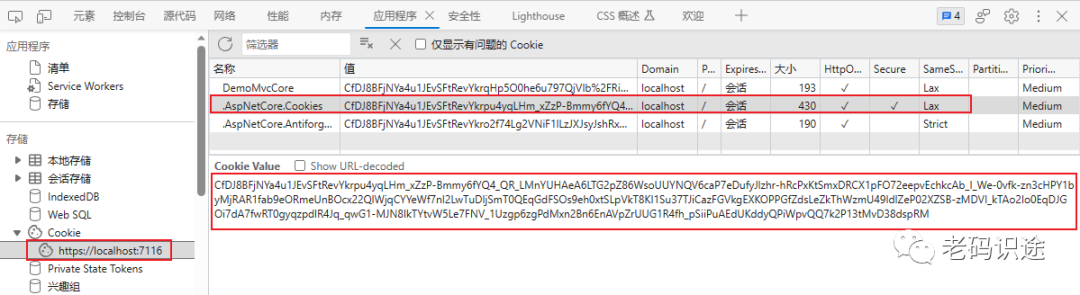
授权区分
namespace Microsoft.AspNetCore.Authorization
{
//
// 摘要:
// Specifies that the class or method that this attribute is applied to requires
// the specified authorization.
[AttributeUsage(AttributeTargets.Class | AttributeTargets.Method, AllowMultiple = true, Inherited = true)]
public class AuthorizeAttribute : Attribute, IAuthorizeData
{
//
// 摘要:
// Initializes a new instance of the Microsoft.AspNetCore.Authorization.AuthorizeAttribute
// class.
public AuthorizeAttribute();
//
// 摘要:
// Initializes a new instance of the Microsoft.AspNetCore.Authorization.AuthorizeAttribute
// class with the specified policy.
//
// 参数:
// policy:
// The name of the policy to require for authorization.
public AuthorizeAttribute(string policy);
//
// 摘要:
// Gets or sets the policy name that determines access to the resource.
public string? Policy { get; set; }
//
// 摘要:
// Gets or sets a comma delimited list of roles that are allowed to access the resource.
public string? Roles { get; set; }
//
// 摘要:
// Gets or sets a comma delimited list of schemes from which user information is
// constructed.
public string? AuthenticationSchemes { get; set; }
}
}