缓存的优点
-
提高应用程序的访问速度
-
适用于不易改变的数据
缓存分类
-
内存缓存:这种方式是将内容缓存到Web服务器内存中,主要适用于单服务器程序,且在服务器重启后,缓存中的数据也会丢失。 -
缓存服务器:对于分布式部署的Web系统,缓存与内存中的方式会造成各个Web服务器中的缓存内容不一致,一般都会有独立的缓存服务器,如Redis,SQL Server等存储缓存的地方。缓存服务器中的内容,不会随着Web服务器的重启而变化。 -
客户端:缓存于客户端一般通过Header实现,也可以通过localStorage,Cookie等方式(暂不讲解)。
内存缓存
1. 添加缓存服务
//内存缓存
builder.Services.AddMemoryCache();
2. 注入缓存接口
private readonly ILogger<HomeController> _logger;
private readonly IMemoryCache _memoryCache;//内存缓存接口
public HomeController(ILogger<HomeController> logger,IMemoryCache memoryCache)
{
_logger = logger;
_memoryCache = memoryCache;
}
3. 获取/设置缓存
public IActionResult Index()
{
if(!_memoryCache.TryGetValue("citys",out List<City> cityList))
{
cityList = GetCitys();
var memoryCacheEntryOptions = new MemoryCacheEntryOptions();
memoryCacheEntryOptions.SetAbsoluteExpiration(TimeSpan.FromSeconds(10));
memoryCacheEntryOptions.RegisterPostEvictionCallback((object key, object value, EvictionReason reason, object state) =>
{
//在被清除缓存时,重新回调,重新填充
_logger.LogInformation("缓存被清除了.");
}, this);
_memoryCache.Set("citys", cityList, memoryCacheEntryOptions);
}
ViewBag.Citys = cityList;
return View();
}
4. 参数说明
-
AbsoluteExpiration 设置绝对过期时间
-
SlidingExpiration 滑动过期时间
-
PostEvictionCallbacks 缓存清除时的回调函数
分布式缓存
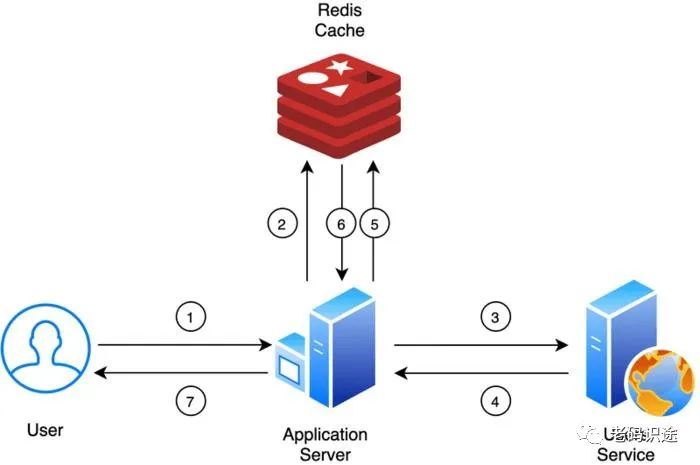
-
无需Sticky Session
-
可扩展,适用于多台Web服务器部署的情况。
-
独立存储,Web服务器重启不会影响缓存
-
性能更好
1. 分布式缓存先决条件
-
对于 Redis 分布式缓存, Microsoft.Extensions.Caching.StackExchangeRedis。
-
对于 SQL Server,请参阅 Microsoft.Extensions.Caching.SqlServer。
-
对于 NCache 分布式缓存, NCache.Microsoft.Extensions.Caching.OpenSource。
2. 环境搭建
-
Redis安装与启动:https://www.cnblogs.com/hsiang/p/14224484.html
-
Redis基础命令:https://www.cnblogs.com/hsiang/p/14269906.html
-
Redis配置文件:https://www.cnblogs.com/hsiang/p/14287098.html
-
Redis事务:https://www.cnblogs.com/hsiang/p/14311126.html
3. 安装依赖包
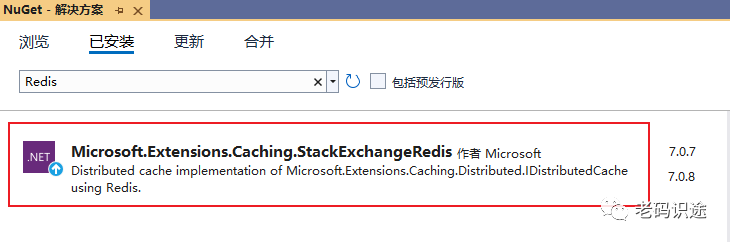
4. 添加分布式缓存服务
//分布式缓存
builder.Services.AddStackExchangeRedisCache(options =>
{
options.Configuration = "192.168.1.6:6379";
options.InstanceName = "redis";
});
5. 注入分布式缓存接口
private readonly IDistributedCache _distributedCache;
public HomeController(ILogger<HomeController> logger ,IDistributedCache distributedCache)
{
_logger = logger;
_distributedCache = distributedCache;
}
6. 获取/设置缓存
public IActionResult Index()
{
var cityList = new List<City>();
var obj = _distributedCache.GetString("citys");
if (string.IsNullOrEmpty(obj))
{
cityList = GetCitys();
DistributedCacheEntryOptions options = new DistributedCacheEntryOptions();
options.SetAbsoluteExpiration(TimeSpan.FromSeconds(60));
obj = JsonConvert.SerializeObject(cityList);
_distributedCache.SetString("citys", obj,options);
}
cityList = JsonConvert.DeserializeObject<List<City>>(obj);
ViewBag.Citys = cityList;
return View();
}
7. 运行测试
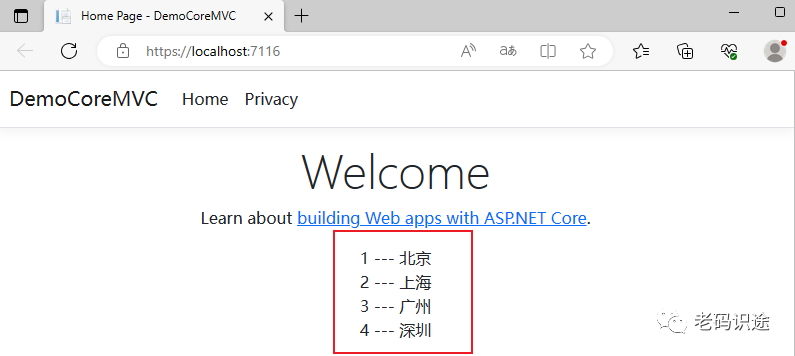
1. 缓存服务器中存储的Key是加了配置的InstanceName前缀。
2. 虽然代码中是通过SetString进行存储,由于存储JSON序列化对象,所以Redis自动识别对象类型为hash。
3. 存储的中文在缓存服务器中是转码后的。
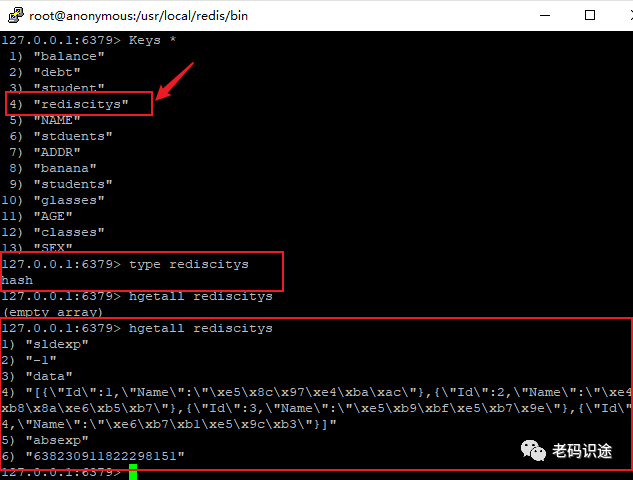