我们通过例子了解这个知识点.
app.MapControllerRoute(
name: "intConstraint",
pattern: "{controller=Home}/{action=Index}/{id:int}");
public IActionResult Check(string id)
{
ViewBag.ValueofId = id ?? "Null Value";
return View();
}
<h1>'Home' Controller, 'Check' View</h1>
<h2>Id value is: @ViewBag.ValueofId</h2>
在上面路由我们能在int后面添加?,指定路由第三段可以接受null值,下面路由约束告诉ASP.NET Core 匹配URL要么是null要么是int值针对第三段
app.MapControllerRoute(
name: "intConstraint",
pattern: "{controller=Home}/{action=Index}/{id:int?}");
2 Range 路由约束
当int类型的url段位于在指定的范围内Range约束能使用匹配,下面路由,我使用了Range 约束:
app.MapControllerRoute(
name: "rangeConstraint",
pattern: "{controller=Home}/{action=Index}/{id:range(5,20)?}");
/Home/Index
/Admin/List/5
/Home/List/15
/Home/List/20
/Home/Index/3
/Home/Index/Hello
/Home/Index/21
下面表给出一些常用的约束
约束 | 描述 |
int | 匹配int值 |
float | 匹配float值 |
decimal | 匹配decimal值 |
datetime | 匹配datetime值 |
double | 匹配double值 |
bool | 匹配true或者false |
length(len) | 匹配值通过使用len指定数量 |
length(min,max) | 匹配值的长度在min和max(包含最小和最大值) |
max(val) | 匹配int的值少于val |
min(val) | 匹配int的值大于val |
range(min,max) | 匹配int的值在min和max之间 (包含最小值和最大值) |
alpha | 匹配仅仅包含字母(A-Z, a-z) |
regex | 匹配值通过正则表达式定义 |
4 Regex 路由约束
Regex路由约束使用正则表达式匹配url,相比别的约束具有更好灵活性,我们能使用正则表达式创建不同的规则,我们在下面路由中使用了正则表达式,针对controller参数
app.MapControllerRoute(
name: "regexConstraint",
pattern: "{controller:regex(^H.*)=Home}/{action=Index}/{id?}");
app.MapControllerRoute(
name: "regexConstraint",
pattern: "{controller:regex(^H.*)=Home}/{action:regex(^Index$|^About$)=Index}/{id?}");
在这个路由中,在url中对controller和action段使用了约束,controller段指定必须H字母开始
action 段指定action段要么是Index要么是About, 因此 如果我打开url- https://localhost:7004/Home/Check/100 ,不能匹配到对应的action方法,因此我们会获取到404错误
/
/Home/Index
/Home/About/
/Home/Index/2
/Home/About/3
Edit/Index/3
/Home/List
5 组合使用路由约束
2 由于alpha约束, 只能使用字母(A-Z, a-z)
app.MapControllerRoute(
name: "combiningConstraint",
pattern: "{controller=Home}/{action=Index}/{id:alpha:regex(^H.*)?}");
/Home/Index/Hello
/Home/Index/Hell
/Home/Index
/Home/Check/HelloJackson
/Home/Index/100
/Home/About/Jackson
/Home/Check/Hello-Jackson
我们能够创建自定义约束用来满足我们客户所需要的特殊场景,让我们通过一个例子来理解客户自定义约束
1. Ram
2. Shiv
3. Krishn
4. Vishnu
5. Brahma
6. Lakshmi
创建自定义约束,根据下面步骤:
1 在应用程序中创建一个文件,名字为CustomConstraint,你可以选择任何你喜欢的名字
2 在文件夹内创建一个类,名字为OnlyGodsConstraint,代码如下:
namespace AspNetCore.RouteConstraint.CustomConstraint
{
public class OnlyGodsConstraint : IRouteConstraint
{
private string[] gods = new[] { "Ram", "Shiv", "Krishn", "Vishnu", "Brahma","Lakshmi" };
public bool Match(HttpContext? httpContext, IRouter? route,
string routeKey, RouteValueDictionary values,
RouteDirection routeDirection)
{
return gods.Contains(values[routeKey]);
}
}
}
using AspNetCore.RouteConstraint.CustomConstraint;
var builder = WebApplication.CreateBuilder(args);
// Add services to the container.
builder.Services.AddControllersWithViews();
builder.Services.Configure<RouteOptions>(options =>
options.ConstraintMap.Add("allowedgods", typeof(OnlyGodsConstraint)));
var app = builder.Build();
// Configure the HTTP request pipeline.
if (!app.Environment.IsDevelopment())
{
app.UseExceptionHandler("/Home/Error");
// The default HSTS value is 30 days. You may want to change this for production scenarios, see https://aka.ms/aspnetcore-hsts.
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseRouting();
app.UseAuthorization();
#region Int约束
//app.MapControllerRoute(
// name: "default",
// pattern: "{controller=Home}/{action=Index}/{id:int}");
#endregion
#region 范围约束
//app.MapControllerRoute(
// name: "rangeConstraint",
// pattern: "{controller=Home}/{action=Index}/{id:range(5,20)?}");
#endregion
#region 路由正则约束
//app.MapControllerRoute(
// name: "regexConstraint",
// pattern: "{controller:regex(^H.*)=Home}/{action=Index}/{id?}");
//app.MapControllerRoute(
// name: "regexConstraint",
// pattern: "{controller:regex(^H.*)=Home}/{action:regex(^Index$|^About$)=Index}/{id?}");
#endregion
#region 组合路由约束
//app.MapControllerRoute(
// name: "combiningConstraint",
// pattern: "{controller=Home}/{action=Index}/{id:alpha:regex(^H.*)?}");
#endregion
#region 客户自定义约束
app.MapControllerRoute(
name: "customerConstraint",
pattern: "{controller=Home}/{action=Index}/{id:allowedgods}"
);
#endregion
app.Run();
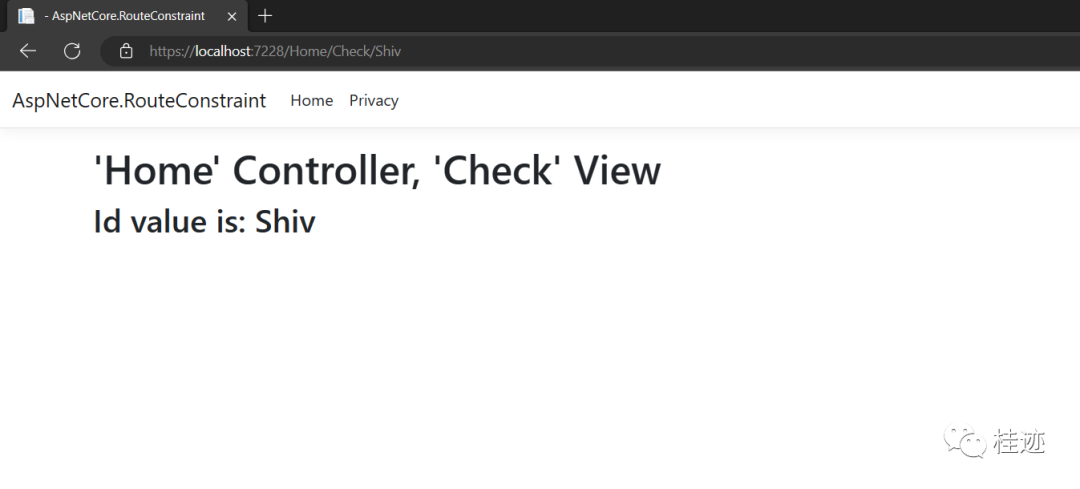
现在,进入- http://localhost:7004/Home/Check/Biden,列表中没有发现Biden,所以不会匹配该URL,我们将获取到404错误
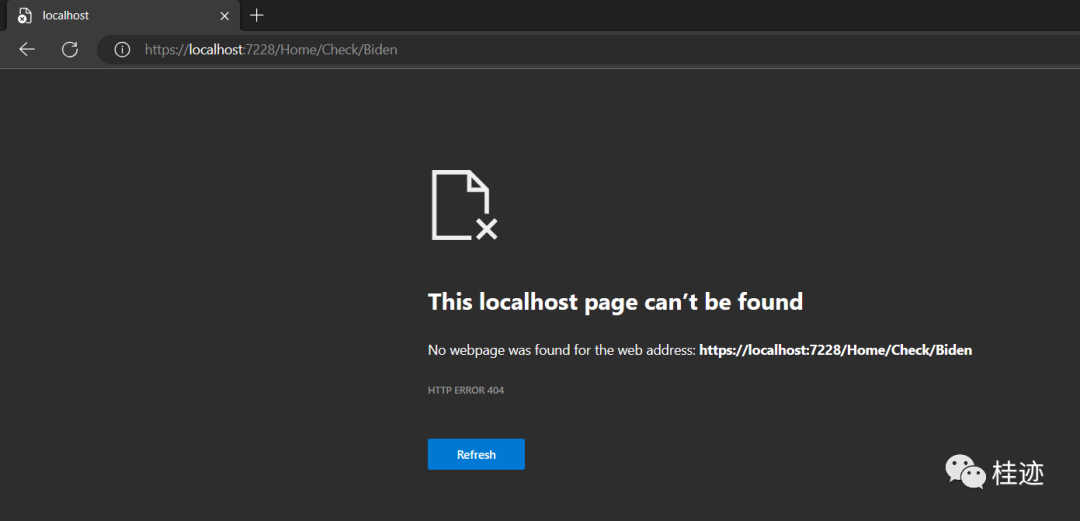
总结:
在这节中,我们学习ASP.NET Core 中的路由约束